Cloud functions
Particle.publishVitals()
Since 1.2.0:
// SYNTAX
system_error_t Particle.publishVitals(system_tick_t period_s = particle::NOW)
Particle.publishVitals(); // Publish vitals immediately
Particle.publishVitals(particle::NOW); // Publish vitals immediately
Particle.publishVitals(5); // Publish vitals every 5 seconds, indefinitely
Particle.publishVitals(0); // Publish immediately and cancel periodic publishing
Publish vitals information
Provides a mechanism to control the interval at which system diagnostic messages are sent to the cloud. Subsequently, this controls the granularity of detail on the fleet health metrics.
Argument(s):
period_s
The period (in seconds) at which vitals messages are to be sent to the cloud (default value:particle::NOW
)particle::NOW
- A special value used to send vitals immediately0
- Publish a final message and disable periodic publishings
- Publish an initial message and subsequent messages everys
seconds thereafter
Returns:
A system_error_t
result code
system_error_t::SYSTEM_ERROR_NONE
system_error_t::SYSTEM_ERROR_IO
Examples:
// EXAMPLE - Publish vitals intermittently
bool condition;
setup () {
}
loop () {
... // Some logic that either will or will not set "condition"
if ( condition ) {
Particle.publishVitals(); // Publish vitals immmediately
}
}
// EXAMPLE - Publish vitals periodically, indefinitely
setup () {
Particle.publishVitals(3600); // Publish vitals each hour
}
loop () {
}
// EXAMPLE - Publish vitals each minute and cancel vitals after one hour
size_t start = millis();
setup () {
Particle.publishVitals(60); // Publish vitals each minute
}
loop () {
// Cancel vitals after one hour
if (3600000 < (millis() - start)) {
Particle.publishVitals(0); // Publish immediately and cancel periodic publishing
}
}
Since 1.5.0:
You can also specify a value using chrono literals, for example: Particle.publishVitals(1h)
for 1 hour.
Sending device vitals does not consume Data Operations from your monthly or yearly quota. However, for cellular devices they do use cellular data, so unnecessary vitals transmission can lead to increased data usage, which could result in hitting the monthly data limit for your account.
NOTE: Diagnostic messages can be viewed in the Console. Select the device in question, and view the messages under the "EVENTS" tab.
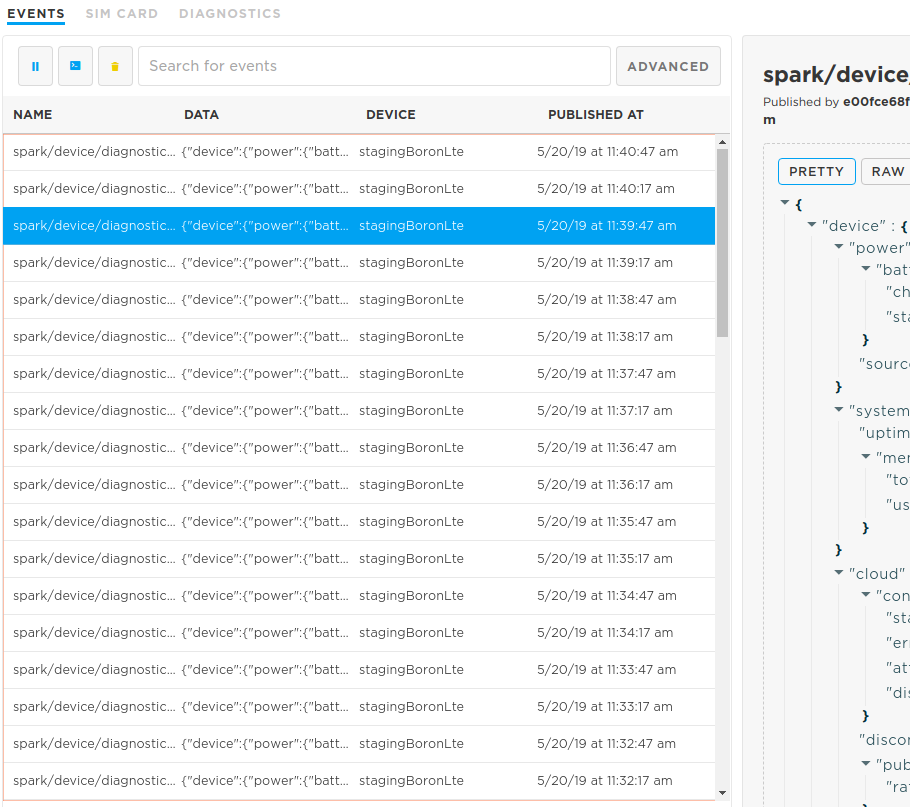
Device vitals are sent:
- On handshake (at most every three days, but can be more frequent if waking from some sleep modes)
- Before an OTA firmware flash if last vitals were sent more than 5 minutes ago
- Under user control from device firmware when using
Particle.publishVitals()
- From the cloud side (API or console) when requested
The actual device vitals are communicated to the cloud in a concise binary CoAP payload. The large JSON event you see in the event stream is a synthetic event. It looks like it's coming from the device but that format is not transmitted over the network connection.
It is not possible to disable the device vitals messages, however they do not count as a data operation.