Firmware libraries
Overview
Libraries are a central part of project development on the Particle platform leveraged by thousands of engineers in our community. Simply stated, a Particle library is a collection of reusable firmware code that can be easily added to one or many Particle projects.
Leveraging high quality libraries to build Internet-connected projects and applications can significantly reduce the risk, time, and cost associated with creating IoT product or device. Libraries also make it possible to easily maintain and reuse common code blocks, as well as incorporate and leverage existing third party libraries from the Particle ecosystem.
Most Arduino libraries are compatible with Particle. We've worked hard to ensure that our firmware API contains all of the most commonly used Arduino functions and firmware commands so that many Arduino libraries can be submitted into the Particle library ecosystem without modification or minimal modification. All of the most popular Arduino libraries are already available through our libraries system, and many others can be easily modified for compatibility.
Particle libraries can include and depend on other Particle libraries. If your library requires another external library as a dependency, it is easy to specify the particular library and even version of the library that your library depends on. A good example is our internet-button
library, which depends on the popular neopixel
library for controlling NeoPixel LEDs. You can learn more about libraries with dependencies in the Library file structure section below.
Kinds of libraries
Public libraries
The vast majority of Particle libraries are developed and maintained by the Particle community and made available for broader use via the Particle libraries ecosystem. All public libraries are available for public consumption through our development tools and via our Libraries API. The availability of such a large number of libraries in a single place makes developing IoT products on the Particle platform fast and simple.
Note that a library may have its own associated open source license that limits or restricts redistribution or commercialization of the library.
Official libraries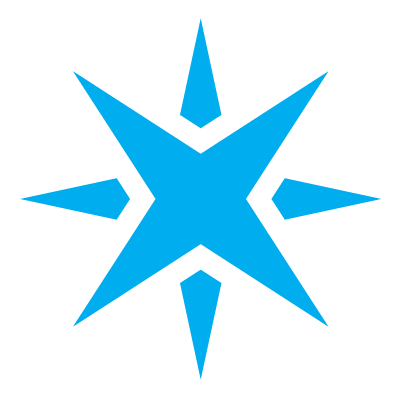
Official libraries are libraries that were created by members of the Particle team and are designed to be used with Particle hardware.
All Particle libraries meet the same quality standards as Verified libraries, and appear in the library list with the Particle logo next to them.
Verified libraries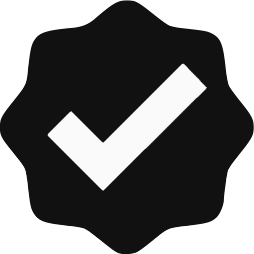
Verified libraries are community-contributed libraries that have been reviewed and confirmed by members of the Particle team to meet the following criteria:
The library is well documented. It contains in-line comments that document proper usage of the library and contains example applications that demonstrate how to use each of the included functions.
The library has been reviewed for quality. The library compiles on all relevant hardware platforms and performs as intended. The library includes testing instructions (
verification.txt
) that anyone can follow to independently verify that the library is working as expected.The library has improved visibility. Verified libraries float to the top of library searches, improving the visibility of the library within the Particle ecosystem.
Private libraries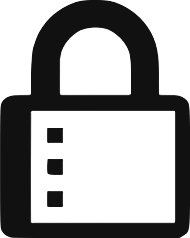
Private libraries are libraries that have been uploaded to the Particle
Device Cloud for reuse with many projects, but are only visible to the individual who created and submitted the library. Private libraries can be published as public libraries at any time by the author of the library using the particle library publish
command.
Workbench and CLI pseudo-libraries
If you are using Particle Workbench or the Particle CLI, you may also want to store shared code across firmware or products using pseuo-libraries in a source code control system like Github.
This allows private sharing of code between multiple users (unlike private libraries), along with robust change logging and version control.
This technique is not available in the Web IDE, however we also do not recommend the Web IDE for product development.
See Workbench pseudo-libraries, below, for more information.
Library file structure
Overview
Every Particle library complies with the following file structure that will be automatically generated when a new library is initialized:
MyLibrary/
examples/
usage/
usage.ino
src/
MyLibrary.cpp
MyLibrary.h
library.properties
README.md
LICENSE
examples
is the folder that contains the example applications that reference your library with one example per directory. If your library controls LEDs, for example, you should include an example called examples/control/control.ino
that demonstrates how someone could use the library in a typical application.
src
is the folder that contains the actual library files (.cpp
and .h
files) that define the library's behavior. Everything in the src
folder will be compiled when a user adds the library to their project and compiles it. You can add subfolders to src
if you have many files in your library.
library.properties
includes descriptive information about your library (title, description, version, author, license), and also specifies any other libraries that your library depends on. Libraries dependencies can be tagged at a particular version in this file.
README.md
provides instructions for library creators on creation and usage.
LICENSE
or LICENSE.txt
is the file that defines the license that the public library is distributed with. All libraries in Particle's library ecosystem must include an associated license.
library.properties fields
name Name of the library. The name must be unique, so there aren't 2 libraries with the same name. It will be the name of the directory as well as the name of main
.cpp
and.h
file. Note that library names must be unique across both public and private libraries. Thus you can get an error uploading a library whose name you cannot see in the community libraries because someone else has created a private library with that name already.version A Semantic Versioning version number like 1.0.0
author Your name and email like
The Author <author@example.com>
. If there are several authors, separate them with commas.license The acronym for the license this library is released under, such as GPL, MIT, Apache-2.
sentence A one sentence description of the library that will be shown in the library browser.
paragraph A longer description to be shown in the details page of the library. This is always shown after sentence so start with the second sentence of the description.
url The web page that a user wanting more information would visit like a GitHub URL.
repository The git repository like
http://github.com/user/project.git
, if available.architectures A comma-separated list of supported hardware boards that are compatible with your library. If missing or set to
*
, the library will be available for all architectures.dependencies.
Other library that this library depends on, one line per library dependency. The value is the desired version number of the library dependency. whitelist Additional files to include when publishing a library. By default these files are included in a library when publishing:
*.ino
,*.pde
,*.cpp
,*.c
,*.c++
,*.h
,*.h++
,*.hpp
,*.ipp
,*.properties
,*.md
,*.txt
,*.S
,*.a
andLICENSE
.
Project file structure
The extended structure expands on the simple structure, placing all application sources in the src
folder.
myapp/
project.properties
src/
myapp.cpp
An extended project has full support for libraries, supporting both library add
and copied libraries.
An extended project can be created by using the CLI particle project create
command.
Your main application file can be a .cpp or a .ino file.
Projects with pseudo-libraries
It's also possible to include library-like files in the lib
directory. See Workbench pseudo-libraries, below, for more information.
myapp/
project.properties
src/
myapp.cpp
lib/
library1/
src/
library1.cpp
SharedCode/
src/
SharedCode.cpp
particle.include and particle.ignore
The particle.include
and particle.ignore
files provide a way to include or exclude additional files from the cloud compiler. Please note that these files are only for special extras and the default files like the source and header files are handled separately and are not affected by the patterns specified in these two files. The include and ignore files can be located either in the project's root directory or in any of its subdirectories.
When the particle.include file is placed in the root directory, files matching the specified patterns are searched recursively from the root directory. On the other hand, if the particle.include file is placed in a subdirectory, the search for matching files is performed recursively starting from that subdirectory as the base. The system automatically handles duplicates, so there is no need to worry about them.
Similarly, the particle.ignore file follows the same behavior, but instead of including files, it excludes files that match the specified patterns from being sent to the cloud compiler.
Example particle.include
file that includes all .def
files:
**/*.def
Example particle.ignore
file to not upload all files from the test
and docs
directories in a library.
test/**/*.*
docs/**/*.*
Please see this Glob Patterns Reference for doing something more custom.
Asset OTA
Particle Workbench and the Particle CLI will automatically generated bundled assets when the project.properties
file contains an assetOtaFolder
key and a value containing a valid directory.
assetOtaFolder=assets
For example:
myapp/
project.properties
src/
myapp.cpp
assets/
coprocessor.bin
Older file structures
These file structures are currently still supported, but may be deprecated in the future. We recommend using the structure above, with the src
directory and a project.properties
file, for all projects.
Using libraries
Libraries consumption is supported in each of our three primary development tools. Instructions for using libraries can be found in the documentation for each of those tools, linked below:
- Library Search
- Using libraries with the Web IDE
- Using libraries with the Particle Workbench
- Using libraries with the Command Line Interface (CLI)
Contributing libraries
The main steps for contributing a library are preparing the structure, writing the code, testing the examples, uploading a private version and publishing to the public.
Library contribution is currently supported via our Command Line Interface (CLI).
Preparing the structure
There are several ways to start contributing a Particle library.
You can start a brand new library by generating the file structure with the Command Line Interface (CLI) using the particle library create
or downloading the example library and editing it.
If you made a library in the past, you can migrate it to the new format with particle library migrate
.
You can modify an existing library by forking the code from GitHub. You can also download an existing library through the CLI with particle library view <library_name>
. Make sure you move the library to a new folder before modifying it in that case.
You can also start with an existing Arduino library from GitHub. Particle libraries have the same structure as Arduino libraries.
Writing the code
The main sources of the library go into src/lib_name.cpp
and src/lib_name.h
. More complex libraries may use a nested structure within the src/
directory. For example src/subFolder/subhHeader.h
and referenced as #include <subFolder/subHeader.h>
.
Create at least one example .ino
file inside a subfolder of examples
to show people how to use the library.
If your library depends on other libraries you can add those dependencies to library.properties
with particle library add
. For example, since the Internet Button contains NeoPixel LEDs, the InternetButton
library has the line dependencies.neopixel=0.0.10
in library.properties
to indicate this.
List the hardware platforms supported by your library supports to the architectures
field in library.properties
, or use *
for all current platforms.
Review the library.properties
and README.md
and fill in as much information as possible.
If you wish to develop libraries using Particle Workbench, see Developing Particle Libraries with Workbench.
Testing the examples
The best way to ensure people will use your library is to provide good examples.
Once you've written the first draft of the library you can test the examples on hardware you own in the CLI with particle flash <my_device> examples/<example_name>
from the library folder.
Uploading a private version
Once your library is ready to go, you can upload it to Particle in the CLI with particle library upload
from the library folder.
When you upload a new private version you can use the library as usual from your own account in the Web IDE, Particle Workbench, or CLI.
If you find issues, you can upload another private version with the same version number.
When you want to modify an existing library for your own projects only, you can stop after uploading a private version. However if you want other people to be able to use the library go on to publishing it.
Publish to the public
When a version is ready for prime time, simply type particle library publish <my_lib>
in the CLI and it will make the latest private version available to the public.
After this, anybody with a Particle account will be able to use your library! Thank you!
v2 library migration (2017)
Common issues with porting libraries
If you have a library
MyLibrary
be sure to have the filesrc/MyLibrary.h
. This should exist, even if it only includes other header files. The Web IDE will automatically add#include "MyLibrary.h"
to the project .ino file when adding the library.When uploading a new version of a library, all files in the library directory are uploaded. Be careful in case you have files in there you don’t want to upload like test binaries and large PDFs. The .gitignore file is not consulted, but you can use the particle.ignore file to skip files.
You can upload a private version multiple times with the same version number, but once you publish a version to the public you won’t be able to upload with the same version number. If you make a small mistake just increase the version number and upload again.
When porting a library, the library itself must have the source in the
src
directory. If it has files in the top level of the library project, they must be moved into a newsrc
directory.When porting a library, the
name
field inlibrary.properties
must match the directory name. This is not enforced on Arduino, so some libraries have a different name here.You cannot set
#define
variables outside the project, such as using a-D
in a Makefile. These external defines will need to be moved into header file instead.You also cannot set an alternate search path in a library (equivalent to a
-I
in a Makefile). Thesrc
directory is added recursively in the main application, but not in libraries.It is not possible to include a pre-compiled binary library (
.a
or.so
) within a Particle library.The Arduino library.properties file does not include the required dependencies. If you are porting a library that depends on other libraries, you will need to add those manually to the Particle library.properties dependencies lines.
Beware of libraries that contain code for other non-Particle hardware platforms. This code can be picked up in the published library and, if large, can cause a timeout while attempting to compile the library, if not an error.
If you are porting code that you want to contribute back upstream, you can surround Particle-specific code with
#ifdef PARTICLE
.If you need to modify a public library for private modifications or to fix a bug, see also Workbench pseudo-libraries, below.
Beware of libraries with LGPL licensing. Due to the way the Particle libraries system works, statically linking the library to the application, the library exception in LGPL licensing does not apply! This means that LGPL (or GPL) licensed libraries cannot be used in closed-source commercial projects.
If a library compile files with
'HAL_Pin_Mode' was not declared in this scope
in Device OS 6.0.0 or later, changeHAL_Pin_Mode
to justpinMode
; this is also backward compatible to older versions of Device OS.If a library compile fails with
error: 'HAL_Pin_Map' was not declared in this scope;
in Device OS 5.0.0 and later, you can fix it like this; the function and structure names changed in later version.
#if defined (SYSTEM_VERSION_v500)
hal_pin_info_t *pinMap = hal_pin_map();
#else
Hal_Pin_Info *pinMap = HAL_Pin_Map();
#endif
If you're having additional issues with library migration or contribution, please feel free to post a message in the community forums.
Modifying a public library
In some cases, you may find that a public library requires a minor modification. For actively maintained libraries, you can just ask the library owner, but in some cases the creator has moved on, at which point you have a few options:
Creating a fork
For many library licenses, you are free to make a derivative version of the library. If the library is stored in Github, you can make a "fork" of the library which maintains a loose connection between the two, making it possible for you to later update your version if the original has been updated, or in some cases the original owner may want to incorporate your changes into the original build.
One issue, however, is the namespace of Particle community libraries is flat, and you cannot upload a new version of a library created by someone else. In this case, the best option is to rename the library, such as by appending a number at the end, or your initials.
You need to update the library name in library.properties as well as the directory name when you upload and publish the library. Also update the README with information about where you obtained the library from (and what version), and what you changed.
Workbench locally modified version
In some cases, you want to modify a library but not publish the changes. If this is the case, see Modifying public libraries, below. Some library licenses, like GPLv3, may not allow using a modified version of a public library in a closed-source project.
Web IDE
It is extremely difficult to use a modified version of a public library in the Web IDE. It's recommended that you use Workbench instead.
If the library is very simple, you can sometimes just directly include the library .cpp and .h files in your Web IDE project, but you may need to also add dependencies, and sometimes paths can be affected, requiring more source changes.
Workbench pseudo-libraries
Using source control
We highly recommend using a source code control system like Github to manage your projects. This assures that you can see all of the changes made over history, who made them, and also provide secure sharing of code. Github provides private repositories in the free plan; Github private repositories can also grant access to a specific list of Github users.
You will generally commit your entire project, with the following notes:
target/
should not be committed, as it contains built binaries and is very large. Include it in your.gitignore
file..vscode/
is normally committed as it contains project settings, but in some cases you may want to commit the base settings once and avoid updating it so your team members won't have any of their private settings overwritten in the future.
This technique can also be used to share a complete project publicly, by making your Github repository public.
Working with public libraries
When you add a library in Workbench using Particle: Install Library it does two things:
- Adds to
project.properties
as a dependency. - Copies the library source into the
lib/
directory at the top of your project.
The local copy in the lib
directory is used when you compile locally. However, if you remove the dependency from project.properties
, then the local copy will also be used for cloud compiles.
We recommend that you:
Include the public library source in your project
lib
directory and store it in source control like Github.Remove the dependency entry from
project.properties
so the behavior will be consistent between local and cloud compiles.
Modifying public libraries
Sometimes you will need to make a small modification to a public library to make it suit your needs, or fix a minor compilation error caused in a new version of the compiler or Device OS. This is easy to do with pseudo-libraries.
- Install the library using Particle: Install Library
- Remove or rename the dependency entry for the library from
project.properties
For example, add disabled_
to the beginning of the dependencies line in project.properties:
name=discombobulator
disabled_dependencies.CellularHelper=0.2.5
Now you are free to make modifications to the library in the lib
directory and these changes will used for local, cloud, and CLI cloud compiles.
Using a 3rd-party library not in the community libraries
Sometimes you will encounter a library that is available in Github, but not in the Particle libraries system. In this case, download or clone the repo into the lib
directory of your project.
Make sure all of the .cpp and .h files are in a src
directory; they cannot be in the top level of the library directory within lib
or in other directories.
See also Common porting issues, above.
Sharing code
You may want to put shared code in a pseudo-library. This keeps it well-organized and separate from your main application. To do this, you create the structure of a library, but not an actual public or private library in the Particle community library system.
MyProject/
project.properties
src/
MyProject.cpp
lib/
SharedCode/
src/
Shared.cpp
Shared.h
In this structure, the pseudo-library SharedCode
is in the lib/
directory. The main requirement is that it contain a src/
directory containing the .cpp and .h files. It can contain subdirectories in src
if desired, and the subdirectories are added to the include search path automatically.
You then have options:
Commit your whole project including
SharedCode
to source control. This does not automate the sharing process but still helps with organization.Use the Github submodule system for managing the shared code. If you think you will be modifying the shared code while working on a project then contributing the changes back up to the shared repository to eventually be incorporated into other projects, this is usually the best option. The biggest annoyance with submodules is that after cloning you must do a manual
git submodule update --init --recursive
to obtain the submodules, and this is not possible when downloading the zip file from the web-based Github.Using Github subrepo feature is the best if you have a set of common code that is only ever consumed by your project, or you are sharing your project publicly as it produces a complete project when downloading the project zip file from the web-based Github.
For more examples, see:
- Tracker Edge uses a mix of source included from the project (like Thermistor) and Github submodules.
- Monitor Edge uses the Github subrepo.